So I used to read Joel On Software quite a bit. A few posts stand out, but one in particular is Making Wrong Code Look Wrong for a few reasons: the problem Joel describes is real, it’s subtle, and the proposed solution is elegant and trivial. Now I’m not going to claim I’m disciplined enough to actually follow it all the time – especially since most of my projects start off as toys where it’s easy enough to keep things right – but I love the idea in theory.
Joel’s simple solution: Hungarian notation. Like many coders before me, I followed the advice from Charles Petzold without knowing why, and like many coders before me I assumed there had to be something I was missing. It wasn’t until Joel’s article explained why Hungarian notation is useful that I learned I was doing it wrong; I instantly saw the value and bought in.
Now unfortunately, Hungarian notation only helps if there are reviewers. It’s obviously possible to write linters to fill the gaps, but that requires more effort than I’m willing to put in. Instead, I’d like to just have the language do the work for me at compile-time. It turns out C++ has an insanely rich type-system, and since objects by themselves add no overhead, this isn’t a completely crazy idea.
struct Width { int value; };
struct Height { int value; };
struct Rectangle {
Rectangle(Width w, Height h) : m_w{w}, m_h{h} { }
Width m_w;
Height m_h;
};
int main() {
Rectangle r{Width{10},
Width{20}}; // compilation error; can't
// convert Width to Height
}
The issue, of course, is scaling. Creating a couple of wrapping types like this is easy, but creating lots becomes a problem. This is especially true when trying to deal with generic code, since somebody might use value
as the underlying variable, and another person could just use v
. Doesn’t matter in most of the code you’ll write, but generic code becomes much more difficult. Operator overloading can solve some of these problems, but now everybody has to get inline
, constexpr
, and noexcept
right, otherwise you’re looking at performance pessimizations.
Thankfully, C++ has templates, which are great for stamping out similar pieces of code. To make the process simpler, I put together some sample code in a new project: biztonsag. The name is the Hungarian word for “safety” per Google Translate, since I don’t actually know Hungarian; I dropped the accent, just to make life simpler on my end, so hopefully no Hungarians are offended.
Now the sample above looks like this:
#include <biztonsag/orderable.hpp>
BTSHN_MAKE_COMPARABLE(int, Width);
BTSHN_MAKE_COMPARABLE(int, Height);
struct Rectangle {
Rectangle(Width w, Height h) : m_w{w}, m_h{h} { }
Width m_w;
Height m_h;
};
int main() {
Rectangle r{Width{10},
Width{20}}; // compilation error; can't
// convert Width to Height
}
Width
and Height
are still strongly typed with no implicit conversions, but now they also support operator*
and operator->
, both in const
and non-const
forms. noexcept
and constexpr
are aggressively applied to all the helper code, so there should be no overhead; a static_assert
verifies no memory overhead.
There are two helper macros to do the heavy lifting: BTSHN_MAKE_COMPARABLE
and BTSHN_MAKE_ORDERABLE
–the difference is that COMPARABLE
types support operator==
and operator!=
while ORDERABLE
types support the other comparing operators.
A simplified version of Joel’s safe string example is available on Github, although it’s not commented yet. I haven’t run the code through Godbolt yet to verify there’s no runtime overhead, but I expect the results will be good.
Update — Performance is good
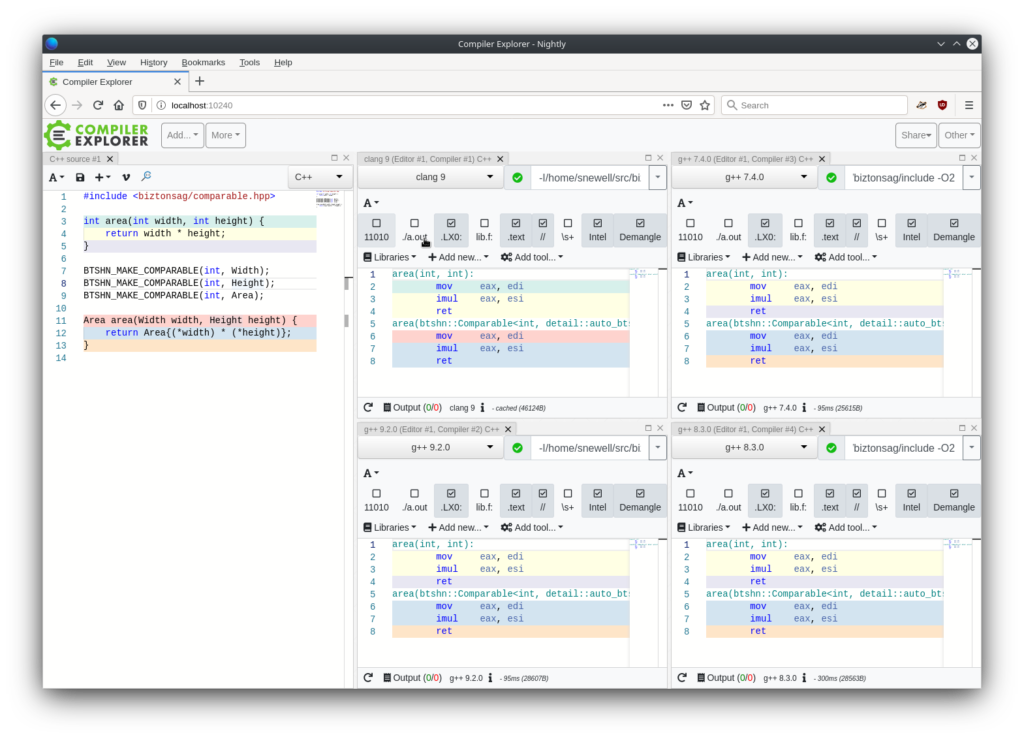
I decided to be non-lazy and actually setup Compiler Explorer locally so I could test biztonsag directly. With four different compilers (gcc‑9.2.0, gcc‑8.3.0, gcc‑7.4.0, and clang‑9.0.1) there’s no runtime overhead once optimizations are enabled. These tests were run on an x86_64 Gentoo Linux system; I don’t have access to other platforms to test.